实现JS的示例调用效果
2017-05-28 18:01:05 访问(1396) 赞(0) 踩(0)
-
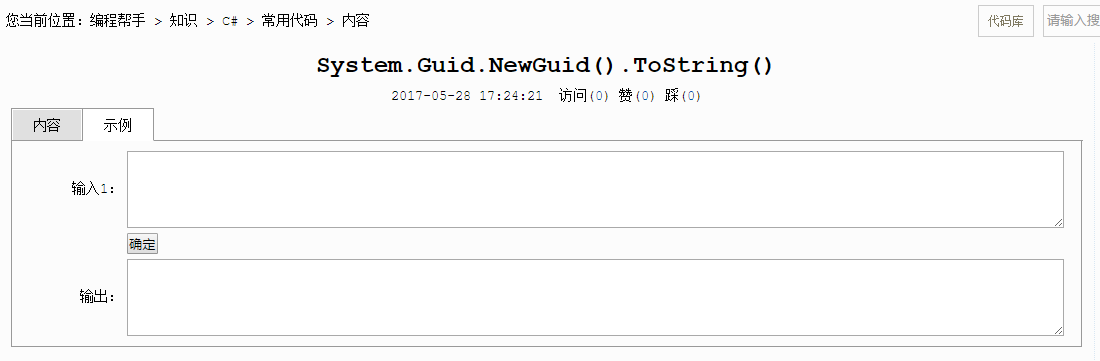
录入交互页面:
实现逻辑:
1、在WebCodeContainer中构建Page
2、JS实现调用
3、ExampleUtilHandler.ashx实现执行
4、SlowX.ExampleUtil.Utils.Util实现逻辑调用
-
<%@ WebHandler Language="C#" Class="ExampleUtilHandler" %>
using System;
using System.Web;
public class ExampleUtilHandler : IHttpHandler {
public void ProcessRequest (HttpContext context) {
context.Response.ContentType = "text/plain";
string theResult = null;
try
{
theResult = ToResult(context, null);
}
catch (Exception err)
{
theResult = err.Message;
}
context.Response.ContentEncoding
=
System.Text.Encoding.GetEncoding("gb2312"); // System.Text.Encoding.UTF8;
context.Response.Write(theResult);
}
/// <summary>
///
/// </summary>
/// <param name="sList"></param>
/// <returns></returns>
protected object[] ListStringToObjArray(System.Collections.Generic.List<string> sList)
{
int iCount = sList.Count;
object[] p = new object[iCount];
for (int i = 0; i < iCount; ++i)
{
p[i] = sList[i];
}
return p;
}
/// <summary>
///
/// </summary>
/// <param name="context"></param>
/// <param name="xdbHelper"></param>
/// <returns></returns>
protected string ToResult(HttpContext context, SlowX.DAL.Helpers.DBHelper xdbHelper)
{
SlowX.WebLib.Classes.Items.ExampleUtilItem
eui
=
new SlowX.WebLib.Classes.Items.ExampleUtilItem();
eui.InitByHttpContext(context);
if(eui.PageId ==0)
return "没有对应的页面记录";
SlowX.WebLib.Helpers.SlowXWebLibHelper
swh
=
SlowX.WebLib.Helpers.SlowXWebLibHelper.cInstance;
SlowX.WebLib.Model.UTB_WEB_CODE_PAGE
model
=
swh.CodePageModelGet(eui.PageId,xdbHelper);
if (model == null)
{
return "没有对应的页面记录";
}
SlowX.ExampleUtil.Utils.Util
ut
=
SlowX.ExampleUtil.Utils.Util.instance;
Type utType = ut.GetType();
System.Reflection.MethodInfo mi
=
utType.GetMethod(model.ButtonClick);
if (mi == null)
{
return "没有找到对应的方法";
}
object[] p = ListStringToObjArray(eui.InputList);
object obj = mi.Invoke(ut, p);
if (obj == null)
return "null";
return obj.ToString();
}
public bool IsReusable {
get {
return false;
}
}
}
-
using System;
using System.Collections.Generic;
using System.Text;
using System.Web;
namespace SlowX.WebLib.Classes.Items
{
/// <summary>
/// JS调用例子的内容设置
/// </summary>
[Serializable]
public class ExampleUtilItem
{
/// <summary>
/// JS调用例子的内容设置
/// </summary>
public ExampleUtilItem()
{
}
#region PageId ~ 页面ID
/// <summary>
/// PageId ~ 页面ID
/// </summary>
protected long m_PageId = 0;
/// <summary>
/// PageId ~ 页面ID
/// </summary>
public long PageId
{
get
{
return m_PageId;
}
set
{
m_PageId = value;
}
}
#endregion PageId ~ 页面ID
/// <summary>
/// 输入项
/// </summary>
public List<string> InputList = new List<string>();
/// <summary>
/// 通过str获得long值
/// </summary>
/// <param name="str"></param>
/// <param name="defaultValue"></param>
/// <returns></returns>
public long LongByStr(string str, long defaultValue)
{
if (str == null)
return defaultValue;
if (str.Length == 0)
return defaultValue;
long theResult = 0;
if (long.TryParse(str, out theResult))
return theResult;
return defaultValue;
}
/// <summary>
/// 通过HttpContext构建内容
/// </summary>
/// <param name="hc"></param>
public void InitByHttpContext(HttpContext hc)
{
// 获得页面ID //
PageId = LongByStr(hc.Request.QueryString["pageid"], 0);
if (PageId == 0)
return;
int maxLoop = int.MaxValue - 1;
string str = null;
for (int i = 1; i < maxLoop; ++i)
{
str = hc.Request.QueryString["s" + i.ToString()];
if (str == null)
break;
str = HttpUtility.UrlDecode(str);
InputList.Add(str);
}
}
}
}
-
using System;
using System.Collections.Generic;
using System.Text;
namespace SlowX.ExampleUtil.Utils
{
/// <summary>
///
/// </summary>
public partial class Util
{
/// <summary>
/// 注释转成Label的名称。比如:角色 - 1.超级管理员;2.部门管理员;3.普通用户 ==> 输出:角色
/// </summary>
/// <param name="theComment"></param>
/// <returns></returns>
public string CommonToLabel(string theComment)
{
if (theComment == null || theComment.Length == 0)
return "";
int idx = theComment.IndexOf(" - ");
if (idx == -1)
return theComment;
else
return theComment.Substring(0, idx);
}
/// <summary>
/// System.Guid.NewGuid().ToString(str) 操作
/// </summary>
/// <param name="str"></param>
/// <returns></returns>
public string ToGuid(string str)
{
try
{
return System.Guid.NewGuid().ToString(str);
}
catch (Exception err)
{
return "[系统异常]:"+err.Message;
}
}
}
}
-
<table width="100%" cellpadding="0" cellspacing="5" border="0">
<tbody><tr>
<td align="right" width="100px">
输入1:
</td>
<td align="left">
<textarea id="txtPage5_1" name="txtPage5_1" rows="2" cols="20" style="height:75px;width:99%;"></textarea>
</td>
</tr>
<tr>
<td align="right" width="100px">
</td>
<td align="left">
<input type="button" id="btn5_Run" name="btn5_Run" value="确定" onclick="AjaxExampleUtilClick('',5,1)">
</td>
</tr>
<tr>
<td align="right" width="100px">
输出:
</td>
<td align="left">
<textarea id="txtPage5_Output" name="txtPage5_Output" rows="2" cols="20" style="height:75px;width:99%;"></textarea>
</td>
</tr>
</tbody></table>
-
// 实现Ajax的执行 //
function AjaxExampleUtilClick(vPhyPath, pageId, inputNumber) {
var ajaxUrl = vPhyPath + "/Ajax/Common/ExampleUtilHandler.ashx?pageid=" + pageId;
var i = 0;
var theCtrl = null;
for (i = 1; i <= inputNumber; ++i) {
theCtrl = document.getElementById("txtPage" + pageId + "_" + i);
ajaxUrl += "&s" + i + "=" + escape(theCtrl.value);
}
$.ajax({
cache: false,
async: true,
url: ajaxUrl,
type: "post",
success: function(data) {
var outputCtrl = document.getElementById("txtPage" + pageId + "_Output");
if (outputCtrl != null)
outputCtrl.value = data;
}
});
}
上一条:
下一条:
相关评论
发表评论